多项选择题
public class Transfers {
public static void main(String[] args) throws Exception {
Record r1 = new Record();
Record r2 = new Record();
doTransfer(r1, r2, 5);
doTransfer(r2, r1, 2);
doTransfer(r1, r2, 1);
// print the result
System.out.println(”rl = “ + r1.get() +“, r2=” + r2.get());
}
private static void doTransfer(
final Record a, final Record b, final int amount) {
Thread t = new Thread() {
public void run() {
new Clerk().transfer(a, b, amount);
}
};
t.start();
}
}
class Clerk {
public synchronized void transfer(Record a, Record b, int amount){
synchronized (a) {
synchronized (b) {
a.add(-amount);
b.add(amount);
}
}
}
}
class Record {
int num=10;
public int get() { return num; }
public void add(int n) { num = num + n; }
}
If Transfers.main() is run, which three are true?()
A. The output may be “r1 = 6, r2 = 14”.
B. The output may be “r1 = 5, r2 = 15”.
C. The output may be “r1 = 8, r2 = 12”.
D. The code may run (and complete) with no output.
E. The code may deadlock (without completing) with no output.
F. M IllegalStateException or InterruptedException may be thrown at runtime.
相关考题
-
单项选择题
public class TestSeven extends Thread { private static int x; public synchronized void doThings() { int current = x; current++; x = current; } public void run() { doThings(); } } Which is true?()
A. Compilation fails.
B. An exception is thrown at runtime.
C. Synchronizing the run() method would make the class thread-safe.
D. The data in variable “x” are protected from concurrent access problems.
E. Declaring the doThings() method as static would make the class thread-safe.
F. Wrapping the statements within doThings() in a synchronized(new Object()) {} block would make the class thread-safe. -
多项选择题
Which three will compile and rim without exception?()
A. private synchronized Object o;
B. void go() { synchronized() { /* code here */ } }
C. public synchronized void go() { /* code here */ }
D. private synchronized(this) void go() { /* code here */ }
E. void go() { synchronized(Object.class) { /* code here */ } }
F. void go() { Object o = new Object(); synchronized(o) { /* code here */ } } -
多项选择题
public class TestFive { private int x; public void foo() { int current = x; x = current + 1; } public void go() { for(int i=0;i<5;i++) { new Thread() { public void run() { foo(); System.out.print(x + “, “); } }.start(); }}} Which two changes, taken together, would guarantee the output: 1, 2, 3, 4, 5, ?()
A. Move the line 12 print statement into the foo() method.
B. Change line 7 to public synchronized void go() {.
C. Change the variable declaration on line 3 to private volatile int x;.
D. Wrap the code inside the foo() method with a synchronized( this ) block.
E. Wrap the for loop code inside the go() method with a synchronized block synchronized(this) { // for loop code here }.
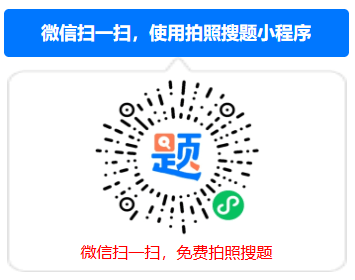